진행 순서
1. 이클립스 설치
2. Project 생성
3. Hello World 작성
4. 실행
5. 자바 실전 도움 예제
************ 시작 ****************
64비트 선택후 다운로드 후 설치
* Java 개발을 할꺼기 때문에 두번째 선택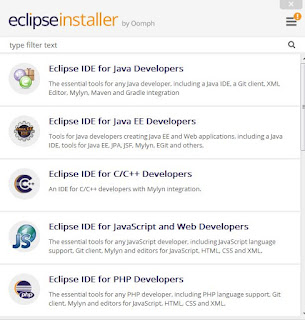
* 설치 경로를 선택후 Install 클릭
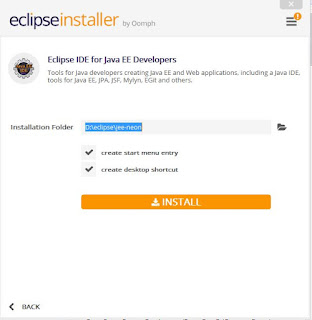
* Launch 클릭 하면 이클립스가 실행됩니다.
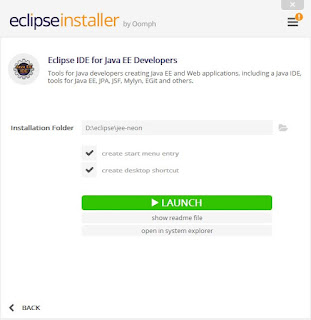
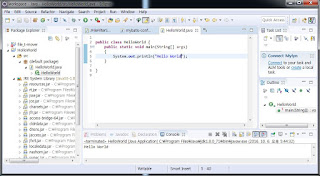
*File - New - Project
- Select a wizard
- Java
- Java Project 선택
public class HelloWorld {
public static void main(String[] args)
{
System.out.println("Hello World");
}
}
eclipse.ini
-Xms256m
-Xmx1024m
이부분을 바꾸어 준다.
Xms1024m 은 Heap영역의 시작크기를 나타내고,
Xmx1024m 은 Heap영역의 최대크기를 나타 냅니다.
int [] num = new int[6];
String strSub;
strSub.substring(4,8);
- charAt은 문자하나를 읽어내지만 substring은 문자열을 읽어 냅니다.
첫번째 인수는 시작지점 문자(반환값에 포함),
두번째 인수는 끝지점에 다음문자(반환값에 포함하지 않는다.)
예) "abcde".substring(1, 3)은 "bc"를 추출해냅니다. 0부터 시작하기 때문입니다.
int numInt = Integer.parseInt(numStr);
int from = 123;
String to = Integer.toString(from);
Calendar oCalendar = Calendar.getInstance( ); // 현재 날짜/시간 등의 각종 정보 얻기
System.out.println("현재 년: " + oCalendar.get(Calendar.YEAR));
System.out.println("현재 월: " + (oCalendar.get(Calendar.MONTH) + 1));
System.out.println("현재 일: " + oCalendar.get(Calendar.DAY_OF_MONTH));
System.out.println(); // 다음줄로 행갈이 하기
System.out.println("현재 시: " + oCalendar.get(Calendar.HOUR_OF_DAY)); // 24시간제
System.out.println("현재 분: " + oCalendar.get(Calendar.MINUTE));
System.out.println("현재 초: " + oCalendar.get(Calendar.SECOND));
System.out.println();
// 12시간제로 현재 시
System.out.print("현재 시: " + oCalendar.get(Calendar.HOUR));
if (oCalendar.get(Calendar.AM_PM) == 0) System.out.println("am");
else System.out.println("pm");
System.out.println("현재 초의 1000분의1초: " + oCalendar.get(Calendar.MILLISECOND));
System.out.println("현재 요일: " + oCalendar.get(Calendar.DAY_OF_WEEK)); // 일요일 = 1
System.out.println("올해 몇 번째 날: " + oCalendar.get(Calendar.DAY_OF_YEAR)); // 1월 1일 = 1
System.out.println("올해 몇 번째 주: " + oCalendar.get(Calendar.WEEK_OF_YEAR)); // 1월 1일은 = 1
System.out.println("이번 달의 몇 번째 주: " + oCalendar.get(Calendar.WEEK_OF_MONTH)); // 첫째 주 = 1
public class Foo {
public static void main(String[] args) {
Calendar oCalendar = Calendar.getInstance( ); // 현재 날짜/시간 등의 각종 정보 얻기
// 1 2 3 4 5 6 7
final String[] week = { "일", "월", "화", "수", "목", "금", "토" };
System.out.println("현재 요일: " + week[oCalendar.get(Calendar.DAY_OF_WEEK) - 1] + "요일");
}
}
Calendar calendar = Calendar.getInstance();
calendar.add(Calendar.DAY_OF_MONTH, -7);
Date date = calendar.getTime();
//date가 일주일전 Date 객체 입니다.
System.out.println(new SimpleDateFormat("yyyy.MM.dd").format(date));
//일주일전 날짜가 찍히겠지요..
참고로 jakarta commons-lang에 보시면 DateUtils 클래스가 있습니다.
DateUtils.addDays(new Date(), -7);
//이렇게 하셔도 됩니다..
String from = "2013-04-08 10:10:10";
SimpleDateFormat transFormat = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
Date to = transFormat.parse(from);
Date from = new Date();
SimpleDateFormat transFormat = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
String to = transFormat.format(from);
System.out.println("같은 날 입니다.");
}
else
{
System.out.println("다른날 입니다.");
return ;
}
public void run()
{
while(1)
{
}
}
public class JsoupP {
public static void main(String args[]) throws IOException {
thPutData thputdata = new thPutData();
//메인이 종료 되어도 스레드는 종료되지 않는다.
thputdata.setDaemon(false);
thputdata.start();
}
}
- 인터럽드로 생성해서 종료하기
자세한 내용은 아래 블러그 이용
http://baeksupervisor.tistory.com/31
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
public class Test01 {
public static void main(String[] args) {
BufferedReader in = null;
try {
URL obj = new URL("http://www.test.co.kr/test.jsp"); // 호출할 url
HttpURLConnection con = (HttpURLConnection)obj.openConnection();
con.setRequestMethod("GET");
in = new BufferedReader(new InputStreamReader(con.getInputStream(), "UTF-8"));
String line;
while((line = in.readLine()) != null) { // response를 차례대로 출력
System.out.println(line);
}
} catch(Exception e) {
e.printStackTrace();
} finally {
if(in != null) try { in.close(); } catch(Exception e) { e.printStackTrace(); }
}
}
}
1. 이클립스 설치
2. Project 생성
3. Hello World 작성
4. 실행
5. 자바 실전 도움 예제
************ 시작 ****************
1. 이클립스 다운
http://www.eclipse.org/downloads/64비트 선택후 다운로드 후 설치
* Java 개발을 할꺼기 때문에 두번째 선택
* 설치 경로를 선택후 Install 클릭
* Launch 클릭 하면 이클립스가 실행됩니다.
2. 실행후 Project 생성
*File - New - Project
- Select a wizard
- Java
- Java Project 선택
* File -> New -> Class 추가
* Name 입력후 Finish
3. 코딩 시작
public class HelloWorld {
public static void main(String[] args)
{
System.out.println("Hello World");
}
}
4. Run(Ctrl + F11)
* 업무시 필요한 자바 개발 정보
폰트명 : Bitstream Vera Sans Mono* 이클립스 실행시 메모리 부족 나오는 경우
이클립스 설치 폴더로 이동eclipse.ini
-Xms256m
-Xmx1024m
이부분을 바꾸어 준다.
Xms1024m 은 Heap영역의 시작크기를 나타내고,
Xmx1024m 은 Heap영역의 최대크기를 나타 냅니다.
* 이클립스 자동정렬 단축키
Ctrl + SHIFT+ F* 자바 배열
선언 및 0으로 초기화int [] num = new int[6];
*지정된 위치만 자르고 싶을때
substring(인수, 인수)String strSub;
strSub.substring(4,8);
- charAt은 문자하나를 읽어내지만 substring은 문자열을 읽어 냅니다.
첫번째 인수는 시작지점 문자(반환값에 포함),
두번째 인수는 끝지점에 다음문자(반환값에 포함하지 않는다.)
예) "abcde".substring(1, 3)은 "bc"를 추출해냅니다. 0부터 시작하기 때문입니다.
* 형변환
* String -> int 변환int numInt = Integer.parseInt(numStr);
int from = 123;
String to = Integer.toString(from);
* 현재 시간 가져오기
import java.util.Calendar;Calendar oCalendar = Calendar.getInstance( ); // 현재 날짜/시간 등의 각종 정보 얻기
System.out.println("현재 년: " + oCalendar.get(Calendar.YEAR));
System.out.println("현재 월: " + (oCalendar.get(Calendar.MONTH) + 1));
System.out.println("현재 일: " + oCalendar.get(Calendar.DAY_OF_MONTH));
System.out.println(); // 다음줄로 행갈이 하기
System.out.println("현재 시: " + oCalendar.get(Calendar.HOUR_OF_DAY)); // 24시간제
System.out.println("현재 분: " + oCalendar.get(Calendar.MINUTE));
System.out.println("현재 초: " + oCalendar.get(Calendar.SECOND));
System.out.println();
// 12시간제로 현재 시
System.out.print("현재 시: " + oCalendar.get(Calendar.HOUR));
if (oCalendar.get(Calendar.AM_PM) == 0) System.out.println("am");
else System.out.println("pm");
System.out.println("현재 초의 1000분의1초: " + oCalendar.get(Calendar.MILLISECOND));
System.out.println("현재 요일: " + oCalendar.get(Calendar.DAY_OF_WEEK)); // 일요일 = 1
System.out.println("올해 몇 번째 날: " + oCalendar.get(Calendar.DAY_OF_YEAR)); // 1월 1일 = 1
System.out.println("올해 몇 번째 주: " + oCalendar.get(Calendar.WEEK_OF_YEAR)); // 1월 1일은 = 1
System.out.println("이번 달의 몇 번째 주: " + oCalendar.get(Calendar.WEEK_OF_MONTH)); // 첫째 주 = 1
* 요일 구하기
import java.util.Calendar;public class Foo {
public static void main(String[] args) {
Calendar oCalendar = Calendar.getInstance( ); // 현재 날짜/시간 등의 각종 정보 얻기
// 1 2 3 4 5 6 7
final String[] week = { "일", "월", "화", "수", "목", "금", "토" };
System.out.println("현재 요일: " + week[oCalendar.get(Calendar.DAY_OF_WEEK) - 1] + "요일");
}
}
* 일주일 전 날짜 구하기
Calendar calendar = Calendar.getInstance();
calendar.add(Calendar.DAY_OF_MONTH, -7);
Date date = calendar.getTime();
//date가 일주일전 Date 객체 입니다.
System.out.println(new SimpleDateFormat("yyyy.MM.dd").format(date));
//일주일전 날짜가 찍히겠지요..
참고로 jakarta commons-lang에 보시면 DateUtils 클래스가 있습니다.
DateUtils.addDays(new Date(), -7);
//이렇게 하셔도 됩니다..
* 형변환(Date)
String to Date
String from = "2013-04-08 10:10:10";
SimpleDateFormat transFormat = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
Date to = transFormat.parse(from);
Date to String
Date from = new Date();
SimpleDateFormat transFormat = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
String to = transFormat.format(from);
*문자열 비교하기
if(sSelectCalendar.equals(sNowCalendar)){System.out.println("같은 날 입니다.");
}
else
{
System.out.println("다른날 입니다.");
return ;
}
*스레드 만들기
class thPutData extends Thread {public void run()
{
while(1)
{
}
}
public class JsoupP {
public static void main(String args[]) throws IOException {
thPutData thputdata = new thPutData();
//메인이 종료 되어도 스레드는 종료되지 않는다.
thputdata.setDaemon(false);
thputdata.start();
}
}
* 스레드 종료하기
- flag 상태값을 수시로 체크하기- 인터럽드로 생성해서 종료하기
자세한 내용은 아래 블러그 이용
http://baeksupervisor.tistory.com/31
* Sleep (5초 쉬기)
Thread.sleep(5 * 1000);* 랜덤 번호로 로또 번호 뽑기
소개 : 배열과 반복문을 이용해 45개의 숫자를 만들고, 랜덤함수를 이용해 배열에 들어있는
1 ~ 45의 숫자를 랜덤하게 섞어준다. 그리고 무작위로 바뀐 배열의 0 ~ 5 자리의 숫자를 출력하는 방식.
class TestJavaFile {
public static void main (String[] args) {
int[] score = new int[45]; //
for(int i=0 ; ilength; i++) {
score[i] = i+1; //1~45개의 숫자 생성
}
for(int i=0 ; i<150 i="" span="">150>
int temp;
int n = (int)(Math.random()* 45);
temp = score[0];
score[0] = score[n];
score[n] = temp; // 0~44의 배열 각 자리수를 무작위로 바꿈
}
System.out.print("Lotto: ");
for(int i=0 ; i<6 i="" span="">6>
System.out.print(score[i]+" "); // 무작위로 바뀐 배열 0~5까지 총 6개의 숫자 뽑기
}
}
}
* 자바에서 URL 호출하기
import java.io.BufferedReader;import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
public class Test01 {
public static void main(String[] args) {
BufferedReader in = null;
try {
URL obj = new URL("http://www.test.co.kr/test.jsp"); // 호출할 url
HttpURLConnection con = (HttpURLConnection)obj.openConnection();
con.setRequestMethod("GET");
in = new BufferedReader(new InputStreamReader(con.getInputStream(), "UTF-8"));
String line;
while((line = in.readLine()) != null) { // response를 차례대로 출력
System.out.println(line);
}
} catch(Exception e) {
e.printStackTrace();
} finally {
if(in != null) try { in.close(); } catch(Exception e) { e.printStackTrace(); }
}
}
}
댓글
댓글 쓰기